The Power of URL Parse in JavaScript
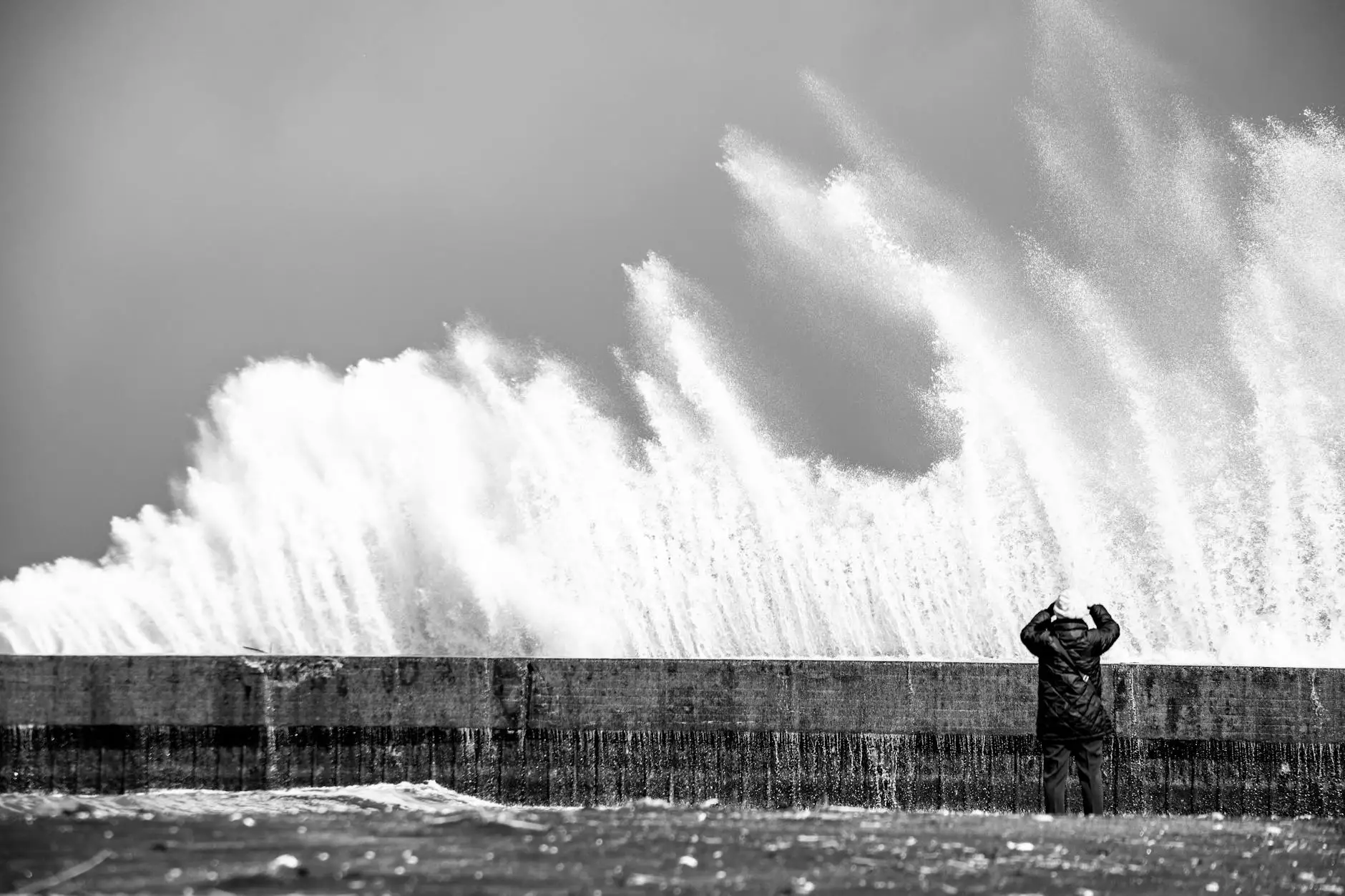
Introduction
As the digital landscape continues to evolve, businesses must adapt to stay ahead in the competitive online market. With web design and software development being vital aspects of a successful online presence, understanding and utilizing effective techniques is necessary. This article explores how URL parsing in JavaScript can play a significant role in enhancing your web design and software development projects.
What is URL Parsing?
URL parsing refers to the process of breaking down a web address or Uniform Resource Locator (URL) into its various components, such as the protocol, domain, path, query parameters, and more. JavaScript provides powerful built-in functionalities that allow developers to parse URLs effortlessly, enabling them to manipulate and extract valuable information from URLs.
The Benefits of URL Parsing in Web Design
URL parsing can greatly improve the functionality and user experience of a website. By extracting specific components from a URL, web designers can dynamically generate content, customize page behavior, and enhance navigation. Let's explore some of the key benefits:
1. Dynamic Content Generation
URL parsing allows web designers to dynamically generate content based on the information contained in the URL. For example, by parsing query parameters, you can display personalized product recommendations, filter search results, or even customize the language or layout of a page based on user preferences.
2. Enhanced Navigation
By parsing the URL, web designers can implement intuitive navigation systems. For instance, extracting path parameters can help create semantic URLs that offer users a clear understanding of the page hierarchy and structure. This enhances user experience and makes the website more search engine friendly.
3. URL Parameter Validation
URL parsing is essential to validate and sanitize user input. Parsing the URL ensures that the provided parameters are in the expected format and range, preventing potential security vulnerabilities such as SQL injection or cross-site scripting (XSS) attacks.
The Role of URL Parsing in Software Development
URL parsing is equally valuable in software development, offering numerous advantages when building web applications, REST APIs, and more. Here are some notable use cases:
1. Routing and Endpoint Handling
URL parsing plays a critical role in handling endpoints and routing requests in web applications. By parsing the URL, developers can identify the specific endpoint requested by the user and trigger the corresponding functionality. This allows for modular and scalable code architecture.
2. Data Extraction and Manipulation
When working with external APIs or parsing data from a web page, URL parsing helps extract valuable information efficiently. Whether it's scraping specific portions of a webpage, extracting metadata, or mapping data to a specific format, URL parsing simplifies the process and ensures accurate data retrieval.
3. SEO and Link Building
URL parsing is closely related to Search Engine Optimization (SEO) and link building strategies. Properly constructed URLs containing relevant keywords can positively impact search engine rankings. By parsing URLs, developers can ensure search engine-friendly URLs, optimizing the chances of higher visibility and organic traffic.
Implementing URL Parsing in JavaScript
Now that we understand the power and benefits of URL parsing, let's take a closer look at how to implement it in JavaScript.
Using the URL API
Modern browsers support the URL API, which provides a convenient way to parse and manipulate URLs. This API makes it easy to access and modify individual components of a URL. Here's an example:
const url = new URL("https://www.example.com/search?q=url+parse+javascript"); console.log(url.protocol); // Output: "https:" console.log(url.hostname); // Output: "www.example.com" console.log(url.pathname); // Output: "/search" console.log(url.search); // Output: "?q=url+parse+javascript"Using Regular Expressions
If you need more flexibility or support in older browsers, regular expressions can be used to parse URLs in JavaScript. Regular expressions allow fine-grained control over the parsing process. Here's an example:
const url = "https://www.example.com/search?q=url+parse+javascript"; const regex = /^([^:]+):\/\/([^/]+)(\/[^?]+)?(\?[^#]+)?(#.*)?$/; const matches = url.match(regex); if (matches) { const protocol = matches[1]; const hostname = matches[2]; const path = matches[3]; const query = matches[4]; const hash = matches[5]; console.log(protocol); // Output: "https" console.log(hostname); // Output: "www.example.com" console.log(path); // Output: "/search" console.log(query); // Output: "?q=url+parse+javascript" console.log(hash); // Output: undefined }Conclusion
URL parsing in JavaScript is an indispensable skill for web designers and software developers alike. By leveraging URL parsing techniques, businesses can optimize their web design projects, improve user experience, handle endpoints efficiently in software development, and enhance search engine visibility through SEO optimization. Understanding and implementing URL parsing effectively empowers businesses to thrive in the ever-evolving digital landscape.
url parse javascript